In this article, we will see the Laravel 10 crud operation step by step.
In this tutorial Laravel 10 crud operation, we will learn about creating, reading, updating, and deleting with the validation rule. Also, we will create a migration for the Laravel 10 crud operation.
CRUD Operation Example with Laravel 10
Step 1: Install Laravel 10 Application For CRUD Operation
Step 2: Database Configuration
Step 3: Create a Migration
Step 4: Add Resource Route
Step 5: Create Controller and Model
Step 6: Create Blade Files
Step 7: Run Laravel 10 Application for CRUD Operation
Step 1: Install Laravel 10 Application For CRUD Operation
In this step, we install Laravel 10 Application for CRUD operation. So, run the following command in the terminal.
composer create-project --prefer-dist laravel/laravel laravel_crud
Step 2: Database Configuration
Now, we do the configuration for setting up the database.
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=laravel_crud
DB_USERNAME=root
DB_PASSWORD=
Step 3: Create a Migration
After that, we create a migration for the products table using the artisan command.
php artisan make:migration create_products_table --create=products
Migration File:
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
return new class extends Migration
{
/**
* Run the migrations.
*/
public function up(): void
{
Schema::create('products', function (Blueprint $table) {
$table->bigIncrements('id');
$table->string('name')->nullable();
$table->decimal('price', 9, 2);
$table->longText('detail')->nullable();
$table->timestamps();
});
}
/**
* Reverse the migrations.
*/
public function down(): void
{
Schema::dropIfExists('products');
}
};
Now, run the migration by the below command.
php artisan migrate
Step 4: Add Resource Route
Now, we add the resource route in the web.php file.
routes/web.php
<?php
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\ProductController;
/*
|--------------------------------------------------------------------------
| Web Routes
|--------------------------------------------------------------------------
|
| Here is where you can register web routes for your application. These
| routes are loaded by the RouteServiceProvider and all of them will
| be assigned to the "web" middleware group. Make something great!
|
*/
Route::resource('products', ProductController::class);
Step 5: Create Controller and Model
In this step, we create the ProductController using the below artisan command.
php artisan make:controller ProductController --resource --model=Product
Now, we will make changes to the Product Model. add the column’s name to the model.
App/Models/Product.php
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
class Product extends Model
{
use HasFactory;
protected $fillable = [
'id', 'name', 'price', 'detail'
];
}
After changes in the model, we need to write code in the ProductController.php file.
app/Http/Controllers/ProductController.php
<?php
namespace App\Http\Controllers;
use App\Models\Product;
use Illuminate\Http\Request;
class ProductController extends Controller
{
/**
* Display a listing of the resource.
*/
public function index()
{
$products = Product::latest()->paginate(10);
return view('products.index',compact('products'))->with('i', (request()->input('page', 1) - 1) * 10);
}
/**
* Show the form for creating a new resource.
*/
public function create()
{
return view('products.create');
}
/**
* Store a newly created resource in storage.
*/
public function store(Request $request)
{
$request->validate([
'name' => 'required',
'price' => 'required|numeric|between:0.00,99999.99',
'detail' => 'required',
]);
Product::create($request->all());
return redirect()->route('products.index')->with('success','Product created successfully.');
}
/**
* Display the specified resource.
*/
public function show(Product $product)
{
return view('products.show',compact('product'));
}
/**
* Show the form for editing the specified resource.
*/
public function edit(Product $product)
{
return view('products.edit',compact('product'));
}
/**
* Update the specified resource in storage.
*/
public function update(Request $request, Product $product)
{
$request->validate([
'name' => 'required',
'price' => 'required|numeric|between:0.00,99999.99',
'detail' => 'required',
]);
$product->update($request->all());
return redirect()->route('products.index')->with('success','Product updated successfully');
}
/**
* Remove the specified resource from storage.
*/
public function destroy(Product $product)
{
$product->delete();
return redirect()->route('products.index')->with('success','Product deleted successfully');
}
}
Step 6: Create Blade Files
In this step, we will create blade files for the layout and product module.
- resources
-- views
--- layouts
------- index.blade.php
------- head.blade.php
------- header.blade.php
------- footer.blade.php
--- products
------- index.blade.php
------- create.blade.php
------- edit.blade.php
------- show.blade.php
resources/views/layouts/index.blade.php
<!DOCTYPE html>
<html>
<head>
@include('layouts.head')
</head>
<body>
<div class="container" style="margin-top: 15px;">
@include('layouts.header')
@yield('content')
@include('layouts.footer')
</div>
</body>
</html>
resources/views/products/index.blade.php
@extends('layouts.index')
@section('content')
<div class="row">
<div class="col-lg-12 margin-tb">
<div class="pull-left">
<h3>Products list </h3>
</div>
<div class="pull-right">
<a class="btn btn-success" href="{{ route('products.create') }}"> Add Product</a>
</div>
</div>
</div>
@if ($message = Session::get('success'))
<div class="alert alert-success">
<p>{{ $message }}</p>
</div>
@endif
<table class="table table-bordered">
<tr>
<th>No</th>
<th>Name</th>
<th>Price</th>
<th>Details</th>
<th width="280px">Action</th>
</tr>
@foreach ($products as $key => $product)
<tr>
<td>{{ $key + 1 }}</td>
<td>{{ $product->name }}</td>
<td>{{ $product->price }}</td>
<td>{{ $product->detail }}</td>
<td>
<form action="{{ route('products.destroy',$product->id) }}" method="POST">
layouts<a class="btn btn-info" href="{{ route('products.show',$product->id) }}">View</a>
<a class="btn btn-primary" href="{{ route('products.edit',$product->id) }}">Edit</a>
@csrf
@method('DELETE')
<button type="submit" class="btn btn-danger">Delete</button>
</form>
</td>
</tr>
@endforeach
</table>
{!! $products->links() !!}
@endsection
resources/views/products/create.blade.php
@extends('layouts.index')
@section('content')
<div class="row">
<div class="col-lg-12 margin-tb">
<div class="pull-left">
<h3>Add Product</h3>
</div>
<div class="pull-right">
<a class="btn btn-primary" href="{{ route('products.index') }}"> Back</a>
</div>
</div>
</div>
<form action="{{ route('products.store') }}" method="POST">
@csrf
<div class="row">
<div class="col-xs-12 col-sm-12 col-md-12">
<div class="form-group">
<strong>Product Name:</strong>
<input type="text" name="name" class="form-control" placeholder="Enter Product Name">
@if ($errors->has('name'))
<span class="error" style="color: #ff5555;">{{ $errors->first('name') }}</span>
@endif
</div>
</div>
<div class="col-xs-12 col-sm-12 col-md-12">
<div class="form-group">
<strong>Product Price:</strong>
<input type="text" name="price" class="form-control" placeholder="Enter Product Price">
@if ($errors->has('price'))
<span class="error" style="color: #ff5555;">{{ $errors->first('price') }}</span>
@endif
</div>
</div>
<div class="col-xs-12 col-sm-12 col-md-12">
<div class="form-group">
<strong>Product Detail:</strong>
<textarea class="form-control" style="height:150px" name="detail" placeholder="Enter Product Detail"></textarea>
@if ($errors->has('detail'))
<span class="error" style="color: #ff5555;">{{ $errors->first('detail') }}</span>
@endif
</div>
</div>
<div class="col-xs-12 col-sm-12 col-md-12 text-center">
<button type="submit" class="btn btn-primary">Submit</button>
</div>
</div>
</form>
@endsection
resources/views/products/edit.blade.php
@extends('layouts.index')
@section('content')
<div class="row">
<div class="col-lg-12 margin-tb">
<div class="pull-left">
<h2>Edit Product</h2>
</div>
<div class="pull-right">
<a class="btn btn-primary" href="{{ route('products.index') }}"> Back</a>
</div>
</div>
</div>
<form action="{{ route('products.update',$product->id) }}" method="POST">
@csrf
@method('PUT')
<div class="row">
<div class="col-xs-12 col-sm-12 col-md-12">
<div class="form-group">
<strong>Product Name:</strong>
<input type="text" name="name" value="{{ $product->name }}" class="form-control" placeholder="Enter Product Name">
@if ($errors->has('name'))
<span class="error" style="color: #ff5555;">{{ $errors->first('name') }}</span>
@endif
</div>
</div>
<div class="col-xs-12 col-sm-12 col-md-12">
<div class="form-group">
<strong>Product Price:</strong>
<input type="text" name="price" value="{{ $product->price }}" class="form-control" placeholder="Enter Product Price">
@if ($errors->has('price'))
<span class="error" style="color: #ff5555;">{{ $errors->first('price') }}</span>
@endif
</div>
</div>
<div class="col-xs-12 col-sm-12 col-md-12">
<div class="form-group">
<strong>Product Detail:</strong>
<textarea class="form-control" style="height:150px" name="detail" placeholder="Detail">{{ $product->detail }}</textarea>
@if ($errors->has('detail'))
<span class="error" style="color: #ff5555;">{{ $errors->first('detail') }}</span>
@endif
</div>
</div>
<div class="col-xs-12 col-sm-12 col-md-12 text-center">
<button type="submit" class="btn btn-primary">Submit</button>
</div>
</div>
</form>
@endsection
resources/views/products/show.blade.php
@extends('layouts.index')
@section('content')
<div class="row">
<div class="col-lg-12 margin-tb">
<div class="pull-left">
<h3> View Product</h3>
</div>
<div class="pull-right">
<a class="btn btn-primary" href="{{ route('products.index') }}"> Back</a>
</div>
</div>
</div>
<div class="row">
<div class="col-xs-12 col-sm-12 col-md-12">
<div class="form-group">
<strong>Product Name:</strong>
{{ $product->name }}
</div>
</div>
<div class="col-xs-12 col-sm-12 col-md-12">
<div class="form-group">
<strong>Product Price:</strong>
{{ $product->price }}
</div>
</div>
<div class="col-xs-12 col-sm-12 col-md-12">
<div class="form-group">
<strong>Product Details:</strong>
{{ $product->detail }}
</div>
</div>
</div>
@endsection
Now, run the following command and start the server.
php artisan serve
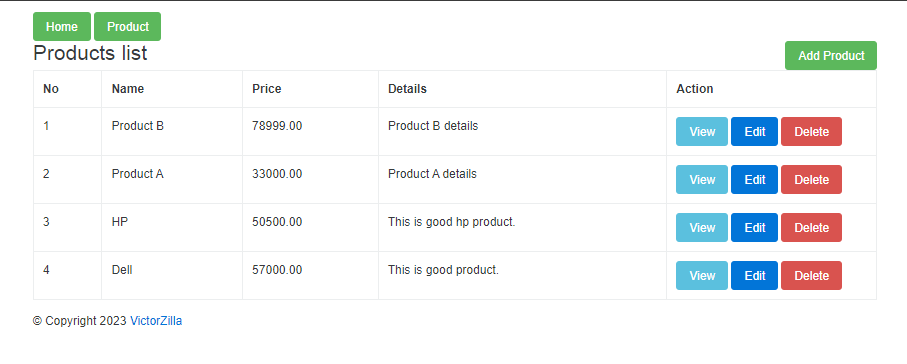
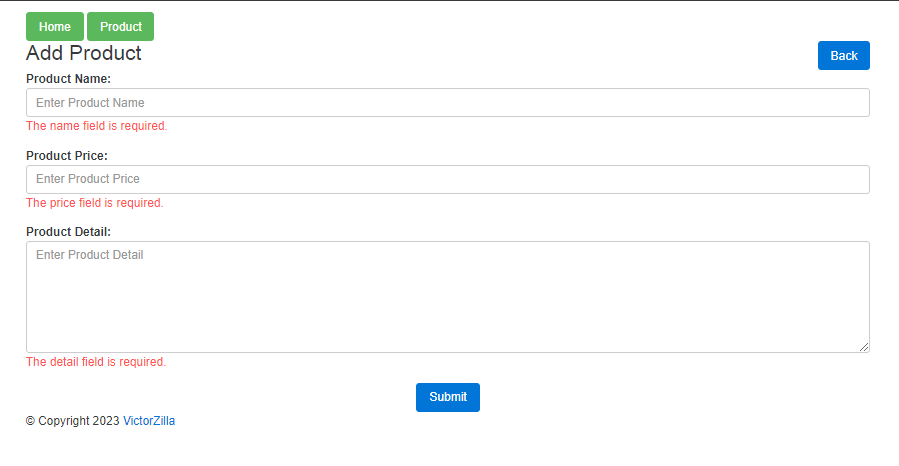
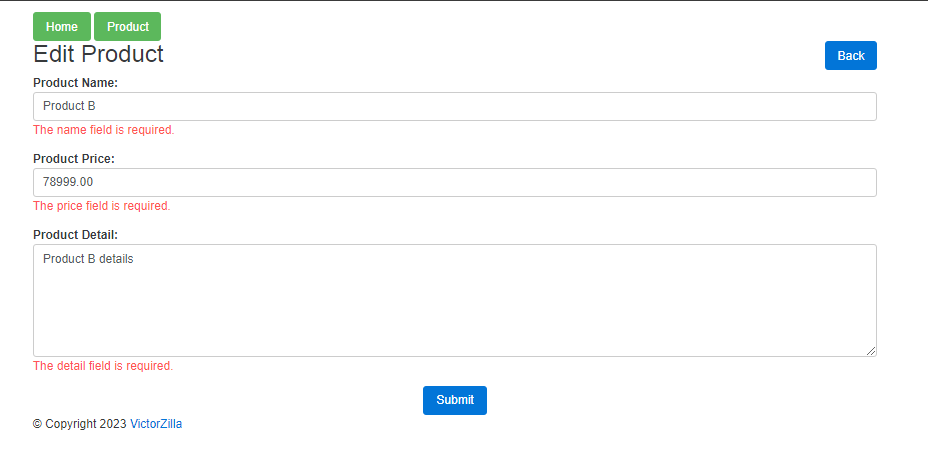